
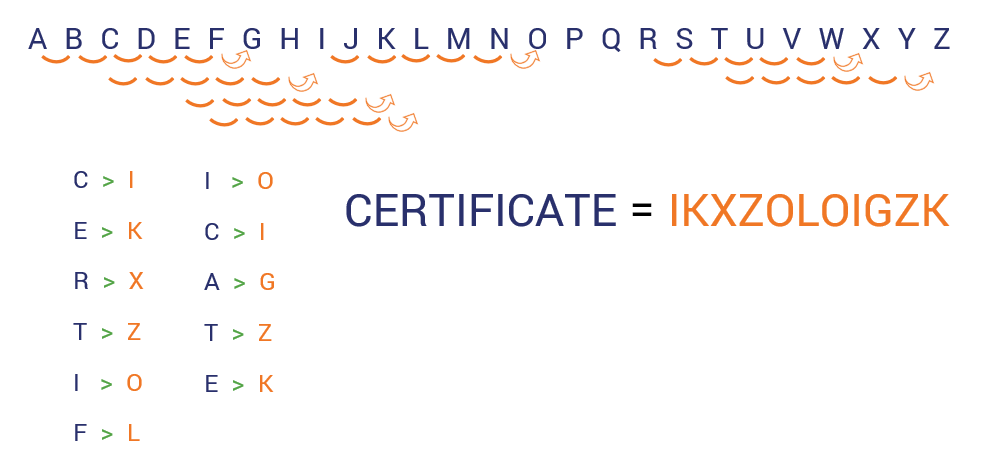
That is how you get the encrypted version of your original sentence. Something like this: public String Encrypt(String message, int key) Step 0: Establish a function that reads in a message and a key. While those are pretty good steps to follow through with, we should think of what we would need to do in code. Step 5: repeat until sentence length is reached. Step 4: Build a new sentence using the new characters in place of the original characters. Note* if the location + key > 26, loop back around and begin counting at one. Step 3: Identify that characters location + the key in the alphabet. Step 2: Find that character’s location within the alphabet. Step 1: Identify the character within the sentence. So, let’s look at the steps necessary to take in order to code this. To implement this code, at least in JAVA, you would need to think through what is actually being done. While this is a very simple example of encryption, it is a perfect project for someone learning to code to practice on. This makes it difficult to read and allows messages to be passed undetected. When this sentence is encrypted using a key of 3, it becomes: Take, for example, a key of 3 and the sentence, “I like to wear hats.” It would take a sentence and reorganize it based on a key that is enacted upon the alphabet. Caesar Cipher is a famous implementation of early day encryption. use for loop for traversing each character of the input stringįor (int i = 0 i "+encryptData(inputStr, shiftKey)) Public static String encryptData(String inputStr, int shiftKey) It is a type of substitution cipher in which each letter in the plaintext is shifted. create encryptData() method for encrypting user input string with given shift key The Caesar cipher is one of the earliest known and simplest ciphers. Public static final String ALPHABET = "abcdefghijklmnopqrstuvwxyz" Each letter is only used once, so if a letter is used twice, only the first occurence is used. The translation alphabet (the letters that are used instead of the ordinary alphabet) starts with the secret key. It is simple type of substitution cipher. ALPHABET string denotes alphabet from a-z The Keyed Caesar cipher is a form of monoalphabetic substitution cipher. Algorithm of Caesar Cipher Caesar Cipher Technique is the simple and easy method of encryption technique. create class CaesarCipherExample for encryption and decryption The method is named after Julius Caesar, who used it in his private correspondence. import required classes and package, if any Caesar cipher: Encode and decode online Method in which each letter in the plaintext is replaced by a letter some fixed number of positions down the alphabet. Let's use the above-discussed steps and implement the code for the Caesar Cipher technique. Depending on the encryption and decryption, we transform each character as per the rule.Traverse input string one character at a time.The input integer should be between 0-25. Take an input integer from the user for shifting characters.Take an input string from the user to encrypt it using the Caesar Cipher technique.We use the following steps to implement the program for the Caesar Cipher technique: We can mathematically represent the encryption of a letter by a shift n in the following way:Įncryption phase with shift n = E n (x) = (x+n)mod 26ĭecryption phase with shift n = D n (x) = (x-n)mod 26 Examples The integer value is known as shift, which indicates the number of positions each letter of the text has been moved down. Based on his name, this technique was named as Caesar Cipher technique.Īn integer value is required to cipher a given text. It substitutes certain letters of the alphabet for others so that words arent immediately recognizable.
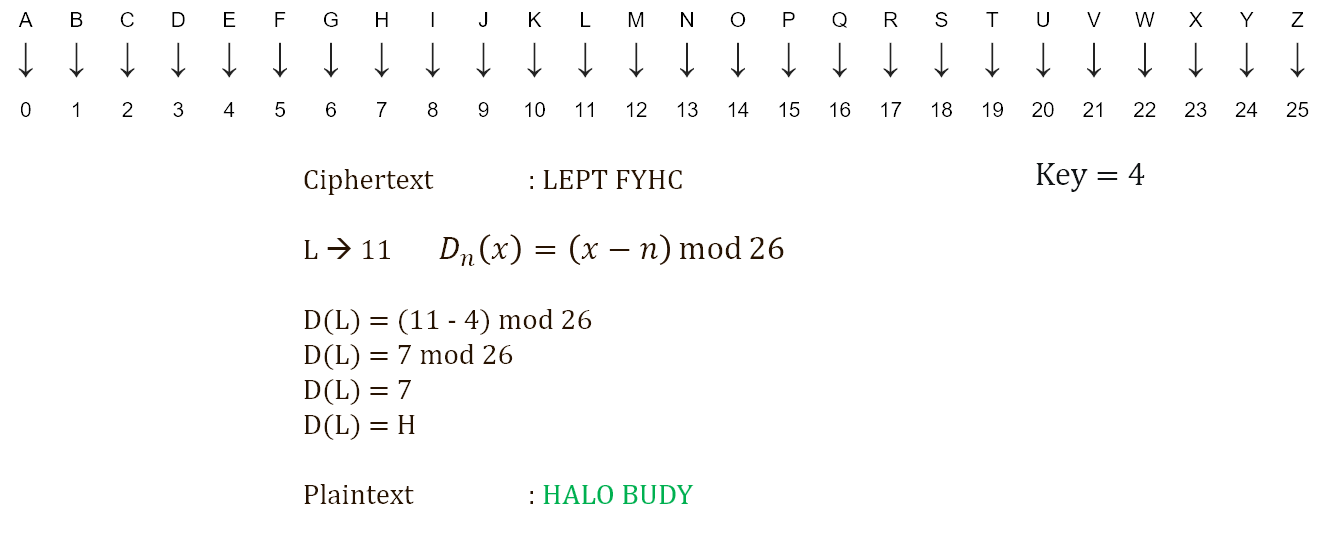
Julius Caesar was the first one who used it for communicating with his officials. The Caesar Cipher is a basic technique for encryption. In this technique, each letter of the given text is replaced by a letter of some fixed number of positions down the alphabet.įor example, with a shift of 1, X would be replaced by Y, Y would become Z, and so on. It is one of the simplest and most used encryption techniques. Next → ← prev Caesar Cipher Program in Java
